一、生成数字加减验证码
1、工具类:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68
|
@Data public class ImageCode {
public String code; public ByteArrayInputStream image; private int wight = 400; private int height = 100;
public static ImageCode getInstance() { return new ImageCode(); }
public ImageCode() { BufferedImage image = new BufferedImage(wight, height, BufferedImage.TYPE_3BYTE_BGR); Graphics graphics = image.getGraphics(); graphics.setColor(new Color(46, 173, 144)); graphics.fillRect(0, 0, wight, height); graphics.setFont(new Font("宋体", Font.PLAIN, 30)); Random random = new Random(); int num1 = random.nextInt(20); int num2 = random.nextInt(20); graphics.setColor(new Color(2, 0, 0)); int Y = 60; graphics.drawString(String.valueOf(num1), wight / 6 * 0 + 50, Y); graphics.drawString("+", wight / 6 * 1 + 50, Y); graphics.drawString(String.valueOf(num2), wight / 6 * 2 + 50, Y); graphics.drawString("=", wight / 6 * 3 + 50, Y); graphics.drawString("?", wight / 6 * 4 + 50, Y); int result = num1 + num2; this.code = result+""; graphics.dispose();
ByteArrayInputStream inputStream = null; ByteOutputStream outputStream = new ByteOutputStream();
try { ImageOutputStream imageOutputStream = ImageIO.createImageOutputStream(outputStream); ImageIO.write(image, "jpg", imageOutputStream);
inputStream = new ByteArrayInputStream(outputStream.toByteArray()); } catch (IOException e) { e.printStackTrace(); } this.image = inputStream; }
}
|
2、controller:
这里存放到redis中了,redis配置自行配。
也可以存放到session中,改为session就行。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41
| @Autowired private StringRedisTemplate redisTemplate;
@GetMapping("/generatorCode") public void generatorCode(HttpServletResponse response) { ImageCode imageCode = ImageCode.getInstance(); String code = imageCode.getCode(); redisTemplate.opsForValue().set("Code",code); ByteArrayInputStream image = imageCode.getImage(); response.setContentType("image/jpg"); byte[] bytes = new byte[1024]; try (ServletOutputStream outputStream = response.getOutputStream()){ while (image.read(bytes) != -1){ outputStream.write(bytes); } } catch (IOException e) { e.printStackTrace(); } }
@GetMapping("/verifyCode") public String verifyCode(String code){ String result = redisTemplate.opsForValue().get("Code"); if (code.equals(result)) { return "success"; } return "error"; }
|
3、测试效果:
先请求生产验证码:这里答案是12
在请求校验接口看是否正确
二、糊涂工具类生产验证码
1、引入依赖
1 2 3 4 5
| <dependency> <groupId>cn.hutool</groupId> <artifactId>hutool-all</artifactId> <version>5.7.21</version> </dependency>
|
2、生产验证码
这里是生成吧验证码生成一张图片,渲染到页面,前端直接拿就行
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20
|
@GetMapping("/getCode") public void getCode(HttpServletResponse response){ LineCaptcha lineCaptcha = CaptchaUtil.createLineCaptcha(116, 36,4,5); redisTemplate.opsForValue().set("Code",lineCaptcha.getCode()); try { ServletOutputStream outputStream = response.getOutputStream(); lineCaptcha.write(outputStream); outputStream.close(); } catch (IOException e) { e.printStackTrace(); } }
|
3、测试效果
三、Happy-captcha生产验证码
官网:https://gitee.com/ramostear/Happy-Captcha?_from=gitee_search
1、导入依赖:
1 2 3 4 5 6
| <dependency> <groupId>com.ramostear</groupId> <artifactId>Happy-Captcha</artifactId> <version>1.0.1</version> </dependency>
|
2、生产验证码:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24
|
@GetMapping("/generateCode") public void generateCode(HttpServletResponse response, HttpServletRequest request){ HappyCaptcha.require(request, response) .style(CaptchaStyle.ANIM) .build() .finish(); }
@GetMapping("/verifyHappyCaptcha") public String verifyHappyCaptcha(String code,HttpServletRequest request){ boolean verification = HappyCaptcha.verification(request, code, true); if (verification) { HappyCaptcha.remove(request); return "success"; } return "error"; }
|
3、测试:
四、easy-captcha生成验证码
依赖:
1 2 3 4 5
| <dependency> <groupId>com.github.whvcse</groupId> <artifactId>easy-captcha</artifactId> <version>1.6.2</version> </dependency>
|
1、生成验证码:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21
|
@GetMapping("/easyGenerateCode") public void easyGenerateCode(HttpServletResponse response, HttpServletRequest request) { ArithmeticCaptcha arithmeticCaptcha = new ArithmeticCaptcha(); arithmeticCaptcha.setLen(2); String content = arithmeticCaptcha.text(); redisTemplate.opsForValue().set("code", content); try { com.wf.captcha.utils.CaptchaUtil.out(arithmeticCaptcha, request, response); } catch (IOException e) { e.printStackTrace(); } }
|
2、效果:
普通验证码:
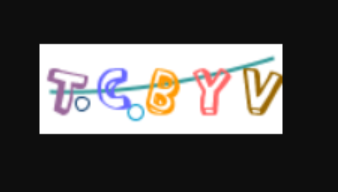
算数验证码:
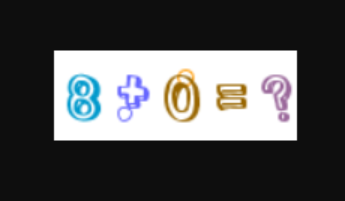
五、Kcaptcha生成验证码
官网:https://gitee.com/
1、引入maven依赖:
1 2 3 4 5
| <dependency> <groupId>com.baomidou</groupId> <artifactId>kaptcha-spring-boot-starter</artifactId> <version>1.0.0</version> </dependency>
|
2、在Controller使用Kaptcha:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27
| @RestController @RequestMapping("/kaptcha") public class KaptchaController {
@Autowired private Kaptcha kaptcha;
@GetMapping("/render") public void render() { kaptcha.render(); }
@PostMapping("/valid") public void validDefaultTime(@RequestParam String code) { kaptcha.validate(code); }
@PostMapping("/validTime") public void validWithTime(@RequestParam String code) { kaptcha.validate(code, 60); }
}
|
3、测试效果:
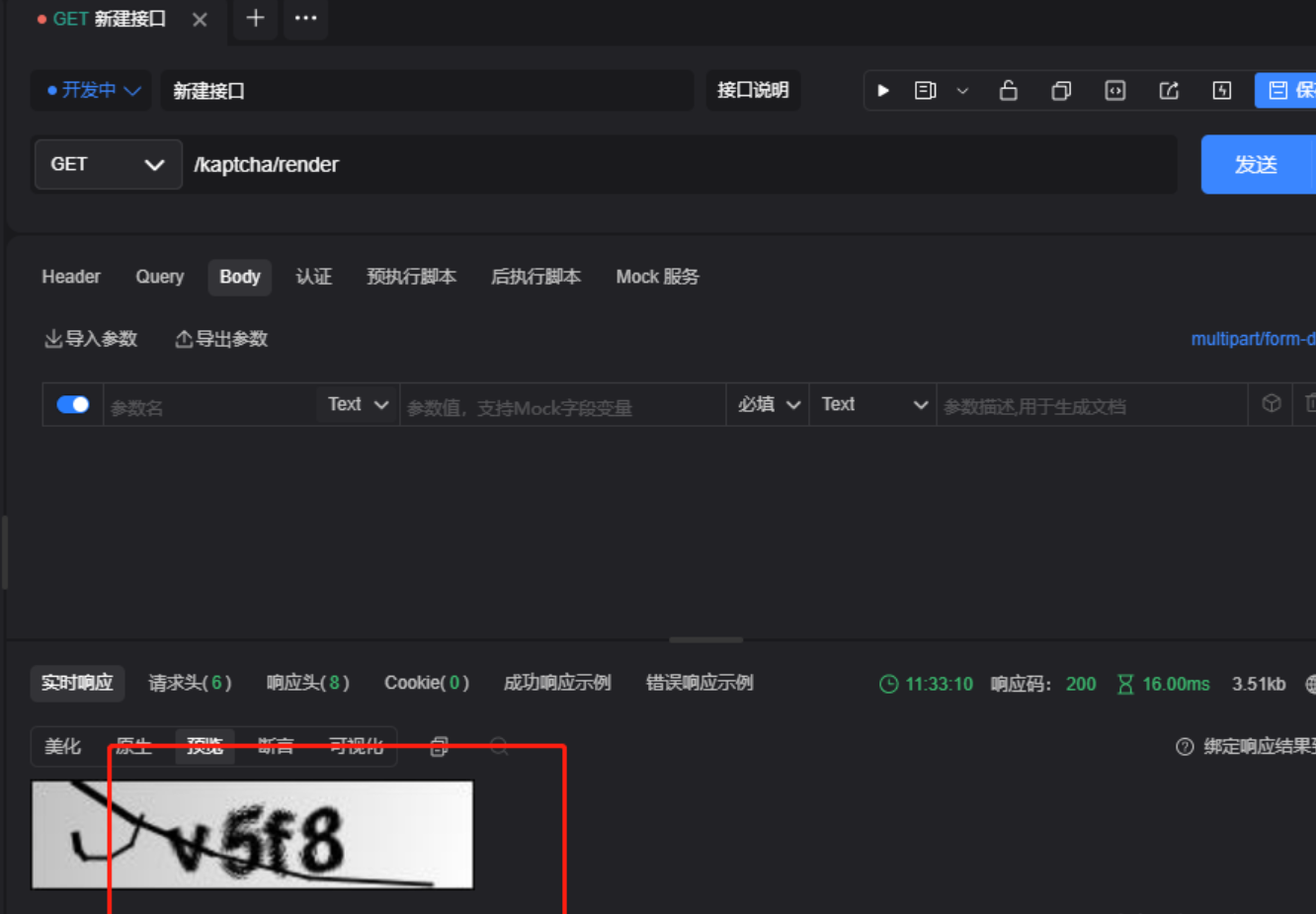